OpenAI File Upload API
I'm trying to create an API for OpenAI's File Upload (https://platform.openai.com/docs/api-reference/files/create).
The request body takes a file object(the file itself) and purpose (string).
This is the code that's in my API call node:
import fs from "fs"; import OpenAI from "openai"; export default async ({apiKey, image_file}: NodeInputs,{ logging }: NodeScriptOptions) : NodeOutput => { const openai = new OpenAI({apiKey}); const file = await openai.files.create({ file: fs.createReadStream(image_file), purpose: "assistants", }); console.log(file); }I only have two inputs (apiKey and image_file) in this node. I've tried passing Buffer into the image_file input, but I get this error:
{ "error": { "code": "ERR_INVALID_ARG_VALUE" }, "label": "API Call", "message": "The argument 'path' must be a string, Uint8Array, or URL without null bytes. Received <Buffer 89 50 4e 47 0d 0a 1a 0a 00 00 00 0d 49 48 44 52 00 00 02 28 00 00 01 6d 08 06 00 00 00 2d 70 91 c7 00 00 00 01 73 52 47 ..." }I tried changing image_file type to string, any, and object, same error. And if I pass the entire File object from the trigger node, I get this error:
{ "error": { "code": "ERR_INVALID_ARG_TYPE" }, "label": "API Call", "message": "The "path" argument must be of type string or an instance of Buffer or URL. Received an instance of Object" }I also tried adding the OpenAI file object into the trigger node's body, and passing the file object into image_file, and I get the same error as above:
{ "error": { "code": "ERR_INVALID_ARG_TYPE" }, "label": "API Call", "message": "The "path" argument must be of type string or an instance of Buffer or URL. Received undefined" }Please advise.
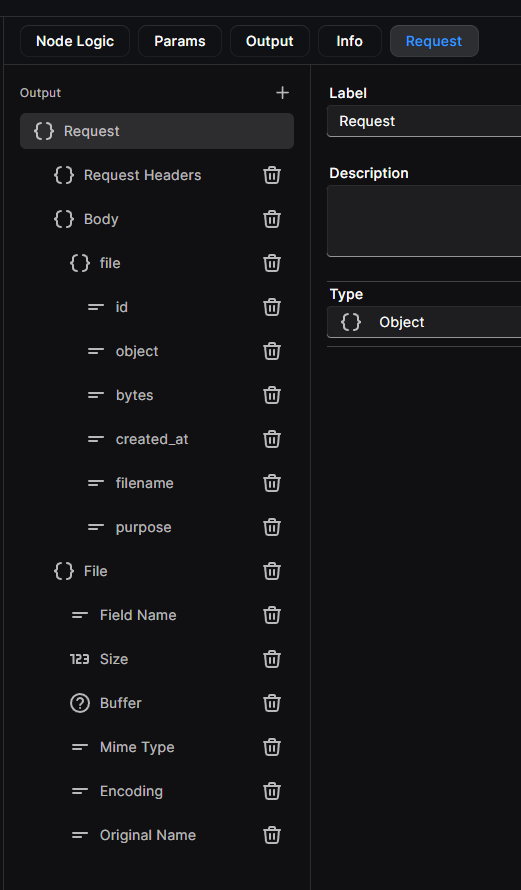
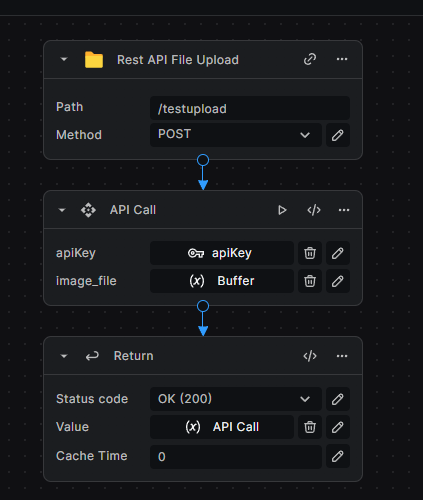
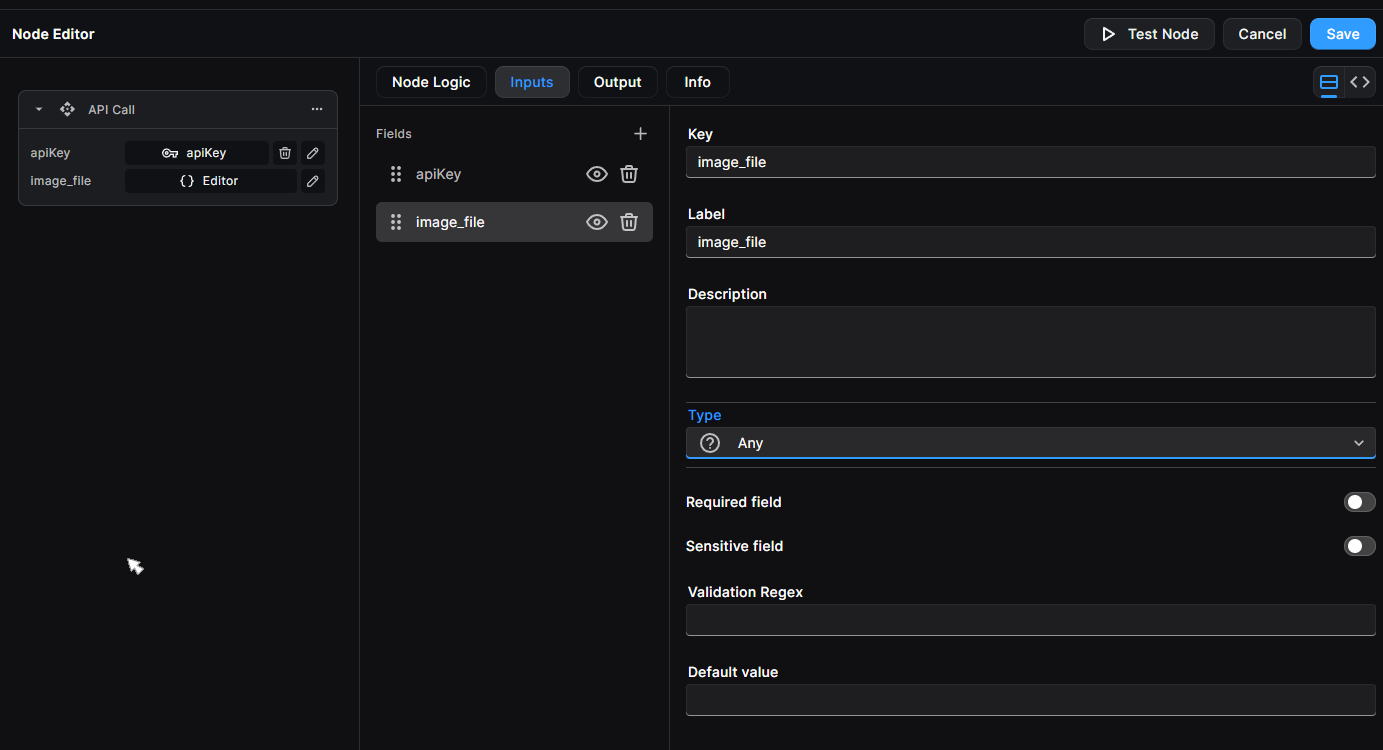
Solution:Jump to solution
@Henry Moses @Sam
i got it to work by writing the buffer to a temp file, and then creating a read stream from the temp file....
9 Replies
have you figured it out?
i am gettig the same error
i have tried multiple ways to solve this but none of them worked...i tried to pre-construct a data-form object, also tried converting the buffer instance into a file object etc etc....but nothing worked...those buildship tutorials are very basic ...
e upload
@Sam no not yet.
i even tried adding "return file;" into the code. And created file object in the output.
nothing is working.
@Harini @Henry Moses could you or one of the experts kindly help us?
Looking at this now!
@Ada
Where is the file being uploaded?
What tool are you using for your frontend?
Because I believe with this API, you can just pass In the URL
@Henry Moses Thanks for looking at this.
The file is uploaded to openai storage.
I'm going to implement it to flutterflow. But right now I'm using postman for testing. So i uploading the file from postman to the API.
I tested the OpenAI API directly with postman before putting it on buildship, and it works with file uploading.
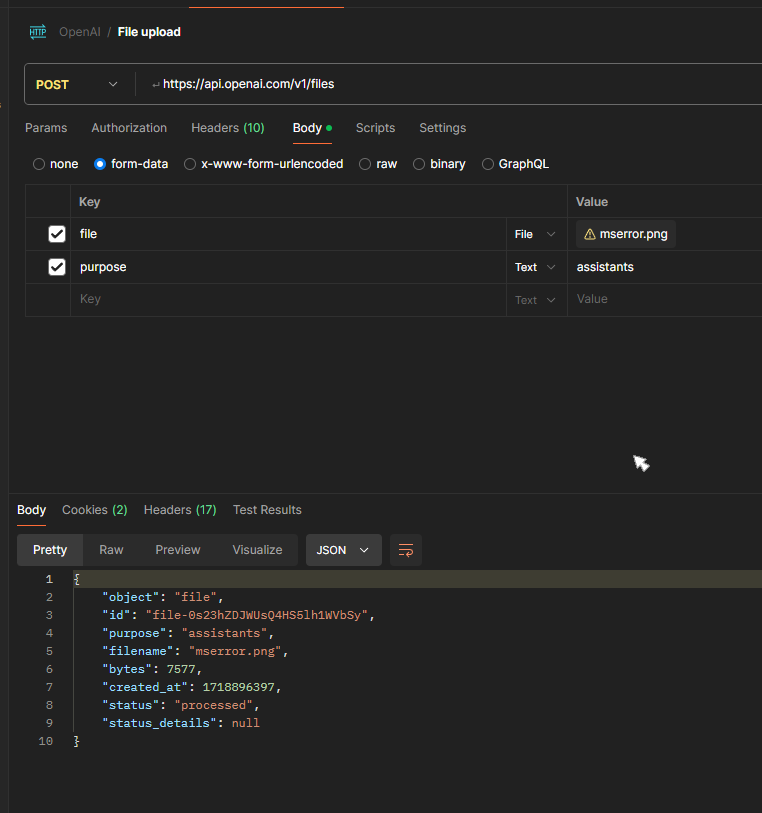
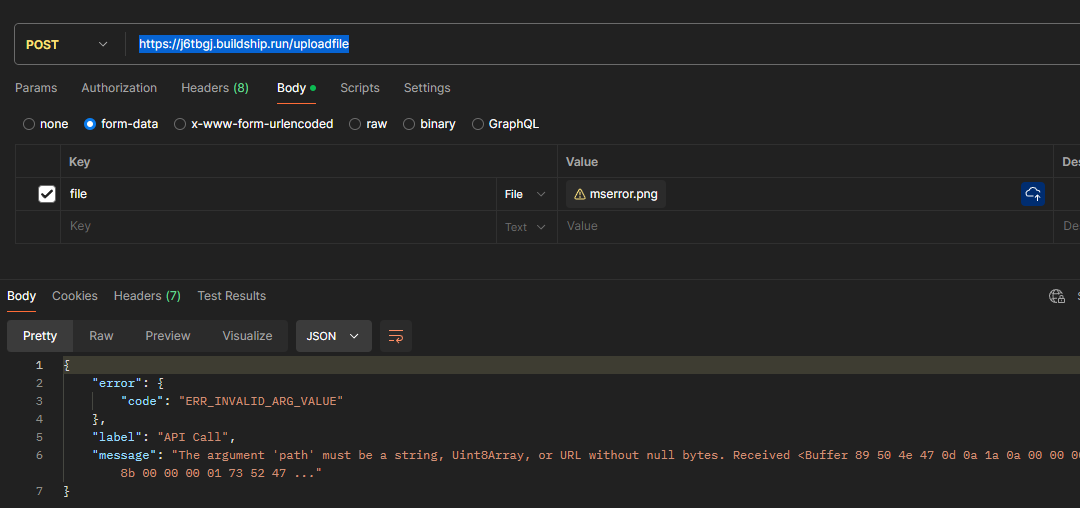
i am using flutterflow...so when the uploaded file from flutterflow reaches buidship through the file upload api node, it becomes a buffer (node.js) instance and is no longer the original file object....this buffer instance of the file can be uploaded to buildship storage or firebase storage but it cannot be sent to OpenAI storage (Add File API), because OpenAI expects the original file object......
I am trying to upload it to OpenAI Files API...We cannot upload a file URL to the files API...
@Henry Moses when i passed the buffer into the return node,
it returns the file contents. For text files, it returns the texts inside. And for image files, it returns a bunch of unreadable texts.
The buffer is NOT file itself, but what's inside the file.
We need to pass in the actual file into the API call directly. How can we do that with the Rest API File upload node?
The OpenAI File Upload API only takes an actual file, not URL or file contents.
So we need to provide either a relative path to the file, or just the file itself. Does that make sense?
Solution
@Henry Moses @Sam
i got it to work by writing the buffer to a temp file, and then creating a read stream from the temp file.
i think this was OpenAI's limitation
Hi @Ada, Thanks for sharing and confirming the solution here.